To finalize the ellipse "library", fuctions are added to draw or fill an ellips which is rotated about the centre of the ellips in stead of rotation about a focal point.
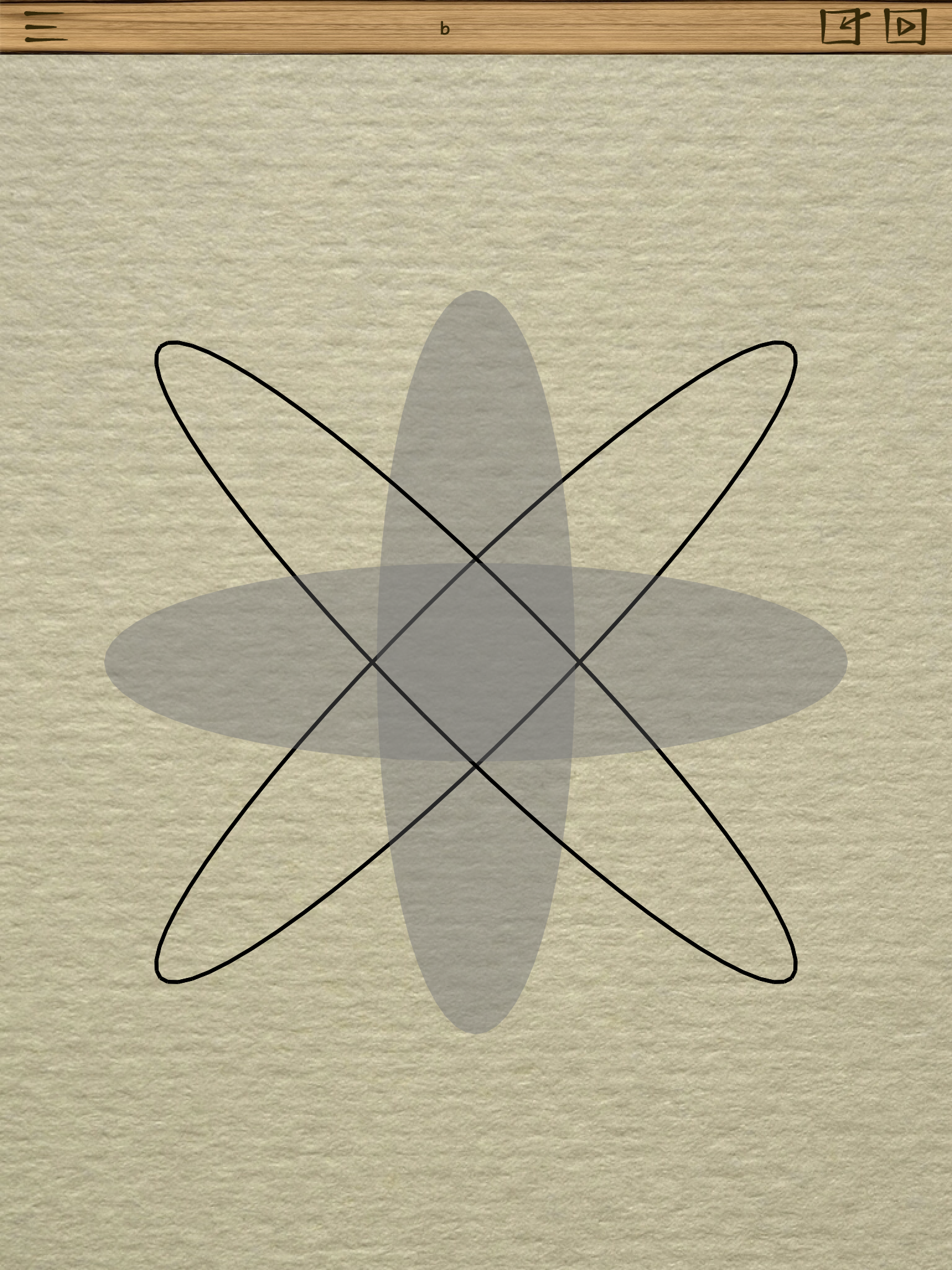
- CAC989EF-2C5C-4AC2-80E2-152FD671711E.png (1.74 MiB) Viewed 7029 times
Code: Select all
graphics ! graphics clear
get screen size sw,sh ! cx=sw/2 ! cy=sh/2
draw color 0,0,0 ! draw size 3
for phi=45 to 315 step 90
draw_ellipse(cx,cy,360,60,phi) ! pause .3
fill_ellipse(cx,cy,300,80,phi+45,.5,.5,.5,.3)
next phi
stop
' draw ellipse rotated counterclockwise with phi degrees
' xc,yc = coordinates of the centre of the ellipse
' ra,rb = horizontal and vertical radii (ra > rb supposed)
' phi = rotation angle in degrees
' option angle in calling code segment is untouched
'
def draw_ellipse(xc,yc,ra,rb,phi)
if sin(90)=1 then oa=1 else oa=0
option angle degrees
dt=6 ! sp=sin(phi) ! cp=cos(phi)
draw to xc+ra*cos(phi),yc-ra*sin(phi)
for t=dt to 360 step dt
st=sin(t) ! ct=cos(t)
draw line to xc+ra*cp*ct-rb*sp*st,yc-ra*sp*ct-rb*cp*st
next t
if oa=1 then option angle degrees else option angle radians
end def
' fill ellipse rotated counterclockwise with phi degrees
' xc,yc = coordinates of the centre of the ellipse
' ra,rb = horizontal and vertical radii (ra > rb supposed)
' phi = rotation angle in degrees
' R,G,B,A = color and alpha info
' option angle in calling code segment is untouched
'
def fill_ellipse(xc,yc,ra,rb,phi,R,G,B,A)
if sin(90)=1 then oa=1 else oa=0
option angle degrees
fill color R,G,B ! fill alpha A
dt=6 ! np=int(360/dt) ! sp=sin(phi) ! cp=cos(phi)
dim x(np+2),y(np+2)
ip=0
for t=0 to 360 step dt
ip+=1 ! st=sin(t) ! ct=cos(t)
x(ip)= xc+ra*cp*ct-rb*sp*st ! y(ip)= yc-ra*sp*ct-rb*cp*st
next t
fill poly x,y
if oa=1 then option angle degrees else option angle radians
end def
' draw ellipse rotated counterclockwise with phi degrees
' xf,yf = coordinates of left focal point
' ra,rb = horizontal and vertical radii (ra > rb supposed)
' phi = rotation angle in degrees
' option angle in calling code segment is untouched
'
def ellipse(xf,yf,ra,rb,phi)
if sin(90)=1 then oa=1 else oa=0
option angle degrees
d_alfa=10
e=sqrt(1-rb*rb/ra/ra)
rho=ra*(1-e*e)/(1-e*cos(-phi))
draw to xf+rho,yf
for alfa=d_alfa to 360 step d_alfa
rho=ra*(1-e*e)/(1-e*cos(alfa-phi))
x=xf+rho*cos(alfa) ! y=yf-rho*sin(alfa)
draw line to x,y
next alfa
if oa=1 then option angle degrees else option angle radians
end def